hdfs的API调用(增删改查)
搭建开发环境
引入依赖
1 2 3 4 5
| <dependency> <groupId>org.apache.hadoop</groupId> <artifactId>hadoop-client</artifactId> <version>2.7.3</version> </dependency>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| <!—指定jdk的版本 --> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>1.8</source> <target>1.8</target> </configuration> </plugin> </plugins> </build>
|
基本配置
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| static FileSystem fileSystem; static { Configuration conf = new Configuration(); conf.set("dfs.replication","3"); try { fileSystem = FileSystem.get(URI.create("hdfs://192.168.120.110:9000"), conf, "root"); } catch (IOException e) { e.printStackTrace(); } catch (InterruptedException e) { e.printStackTrace(); } }
|
1 2 3 4 5 6 7 8 9 10 11
|
public static void makedir() throws IOException { boolean mkdirs = fileSystem.mkdirs(new Path("/bigdata")); if (mkdirs){ System.out.println("创建成功"); }else{ System.out.println("创建失败"); } }
|
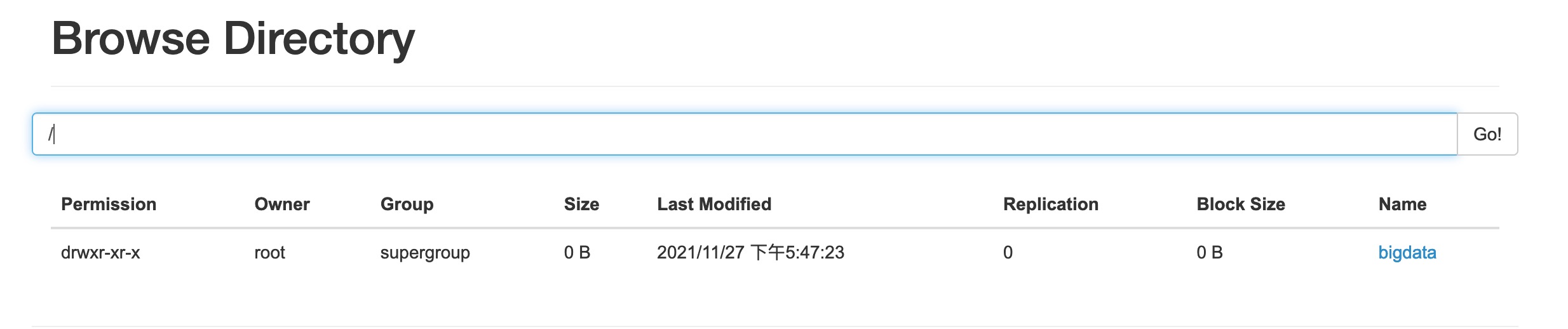
1 2 3 4 5 6 7 8 9
| public static void deldir() throws IOException { boolean delete = fileSystem.delete(new Path("/bigdata")); if (delete){ System.out.println("删除成功"); }else{ System.out.println("删除失败"); } }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
|
public static void alter(){ try { boolean rename = fileSystem.rename(new Path("/bigdata"), new Path("/bigData")); if(rename){ System.out.println("修改成功!!!"); }else{ System.out.println("修改失败!!!"); } } catch (IOException e) { e.printStackTrace(); } }
|
1 2 3 4 5 6
|
public static void upLoad() throws IOException { fileSystem.copyFromLocalFile(new Path("/Users/haikez/Desktop/hadoop学习/hadoop1.7.2.rar"),new Path("/bigData/")); }
|
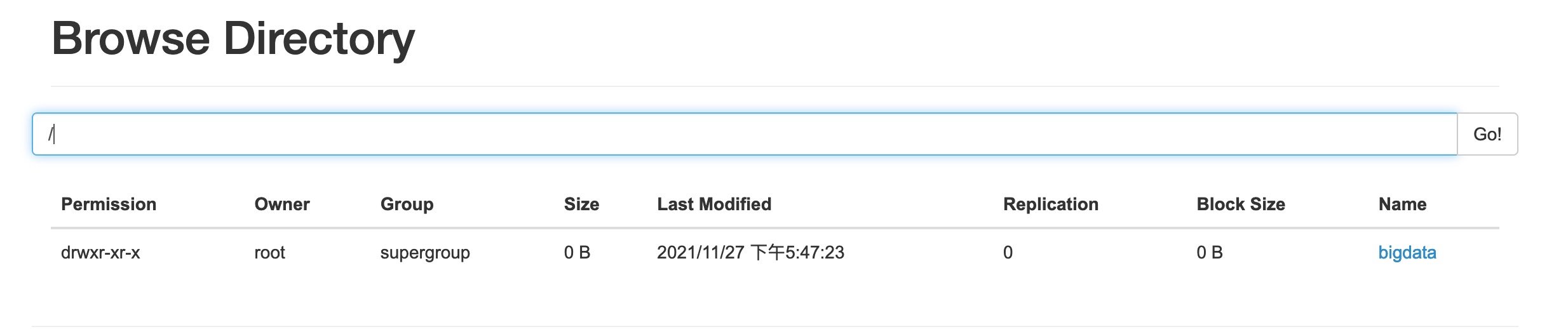
1 2 3 4
| public static void downLoad() throws IOException { fileSystem.copyToLocalFile(new Path("/bigData/hadoop1.7.2.rar"),new Path("/Users/haikez/Desktop/")); }
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
|
public static void listStatus() throws IOException { FileStatus[] listStatus = fileSystem.listStatus(new Path("/bigData")); for (FileStatus status : listStatus) { System.out.println("文件大小:"+status.getLen()); System.out.println("文件路径:"+status.getPath()); System.out.println("文件副本数:"+status.getReplication()); System.out.println("block块大小:"+status.getBlockSize()); System.out.println("文件上传时间"+status.getModificationTime()); } }
|
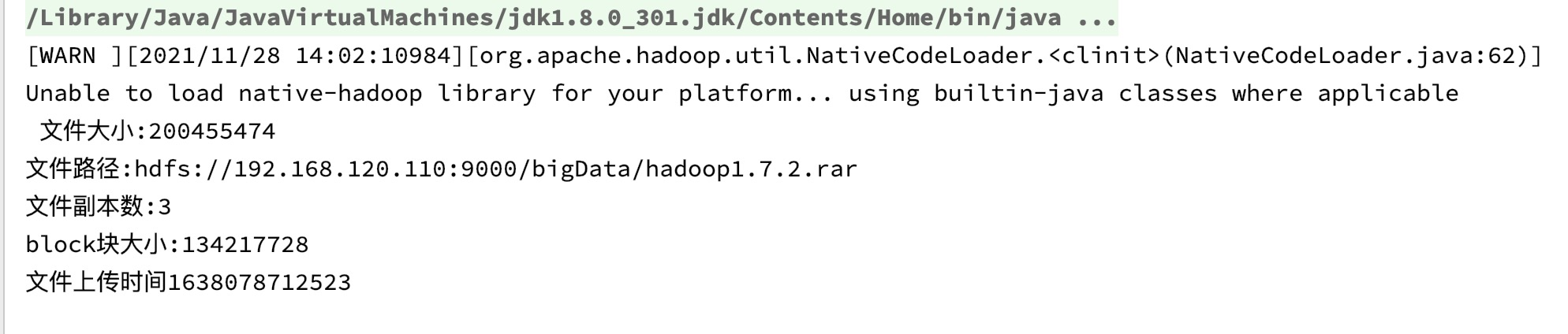
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
|
public static void listFiles() throws IOException { RemoteIterator<LocatedFileStatus> listFiles = fileSystem.listFiles(new Path("/"),true); while (listFiles.hasNext()){ LocatedFileStatus fs = listFiles.next(); System.out.println("文件大小:"+fs.getLen()); System.out.println("文件路径:"+fs.getPath()); System.out.println("文件副本数:"+fs.getReplication()); System.out.println("block块大小:"+fs.getBlockSize()); System.out.println("文件上传时间"+fs.getModificationTime()); System.out.println("------------"); } }
|
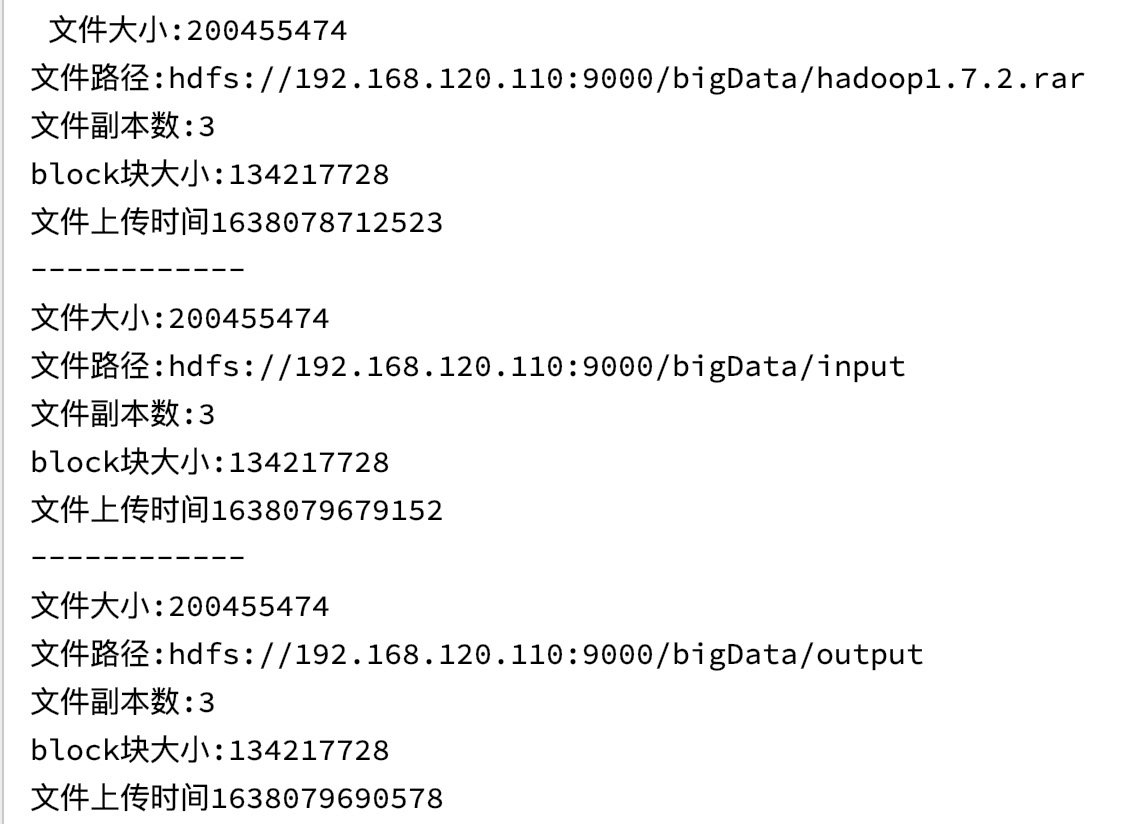
1 2 3 4 5 6 7 8 9 10
| public static void main(String[] args) throws IOException {
listFiles(); }
|
-------------本文结束感谢您的阅读-------------