过滤器与监听器
一、过滤器(Filter)
用于过滤网站的数据;
- 处理中文代码
- 登录验证
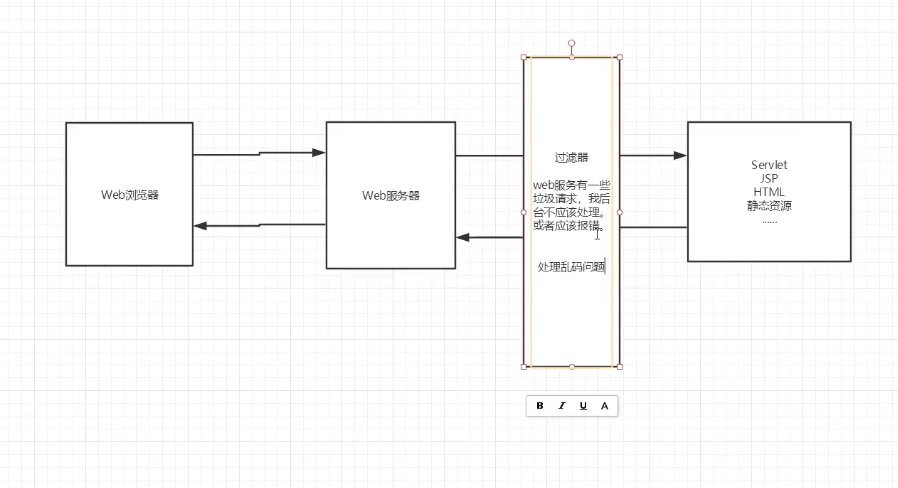
1. 创建过滤器
编写一个过滤器实现Filter接口(注意导包)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26
| public class CharacterEncodingFilter implements Filter {
@Override public void init(FilterConfig filterConfig) throws ServletException { System.out.println("CharacterEncodingFilter初始化"); }
@Override public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException { servletRequest.setCharacterEncoding("utf-8"); servletResponse.setCharacterEncoding("utf-8"); servletResponse.setContentType("text/html;charset=utf-8"); filterChain.doFilter(servletRequest,servletResponse); }
@Override public void destroy() { System.out.println("CharacterEncodingFilter销毁"); } }
|
记住创建过滤器的时候,要链接到下一个过滤器。filterChain.doFilter(servletRequest,servletResponse);(固定不变的代码)
2. 在web.xml中配置过滤器(和servlet类似)
1 2 3 4 5 6 7 8 9 10 11 12
| <filter>
<filter-name>CharacterEncodingFilter</filter-name> <filter-class>com.nichu.filter.CharacterEncodingFilter</filter-class> </filter> <filter-mapping> <filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/hello/*</url-pattern> </filter-mapping>
|
过滤器本质上就是一个servelt程序
二、监听器(Listener)
1. 监测网站在线人数(基于session)
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32
| public class OnlineCountListener implements HttpSessionListener { @Override public void sessionCreated(HttpSessionEvent httpSessionEvent) { ServletContext ctx = httpSessionEvent.getSession().getServletContext(); Integer onlineCount = (Integer) ctx.getAttribute("onlineCount"); if(onlineCount==null){ onlineCount=new Integer(1); }else{ int count=onlineCount.intValue(); onlineCount=new Integer(count+1); } ctx.setAttribute("onlineCount",onlineCount); }
@Override public void sessionDestroyed(HttpSessionEvent httpSessionEvent) { ServletContext ctx = httpSessionEvent.getSession().getServletContext(); Integer onlineCount = (Integer) ctx.getAttribute("onlineCount"); if(onlineCount==0){ onlineCount=new Integer(1); }else{ int count=onlineCount.intValue(); onlineCount=new Integer(count-1); } ctx.setAttribute("onlineCount",onlineCount); } }
|
2. 编写index.jsp页面
1 2 3 4 5 6
| <%@page contentType="text/html; charset=utf-8" %> <html> <body> <h1>当前有<span><%=this.getServletConfig().getServletContext().getAttribute("onlineCount")%></span>人在线</h1> </body> </html>
|
3. 在web.xml中注册监听器
1 2 3 4
| <listener> <listener-class>com.nichu.listener.OnlineCountListener</listener-class> </listener>
|
4. session销毁方法
- 手动销毁 getSession().invalidate();
- 自动销毁 在web.xml中设置超时时间
1 2 3 4
| <session-config>
<session-timeout>1</session-timeout> </session-config>
|