一、使用注解开发
1. 注解在接口上实现
1 2 3 4
| public interface UserMapper { @Select("select * from user") List<User> getUser(); }
|
2. 需要在核心配置文件中绑定接口!
1 2 3 4
| <mappers> <mapper class="com.nichu.dao.UserMapper"/> </mappers>
|
3. 测试
1 2 3 4 5 6 7
| public static void main(String[] args) { SqlSession sqlSession = MybatisUtils.getSqlSession(); UserMapper mapper = sqlSession.getMapper(UserMapper.class); List<User> user = mapper.getUser(); System.out.println(user); sqlSession.close(); }
|
注解开发的
1 2 3 4 5
| 本质:反射机制实现 底层:动态代理! 弊端:使用注解来映射简单语句会使代码显得更加简洁,但对于稍微复杂一点 的语句,Java 注解不仅力不从心,还会让你本就复杂的 SQL 语句更加混乱 不堪。 因此,如果你需要做一些很复杂的操作,最好用 XML 来映射语句。
|
二、Mybatis执行流程
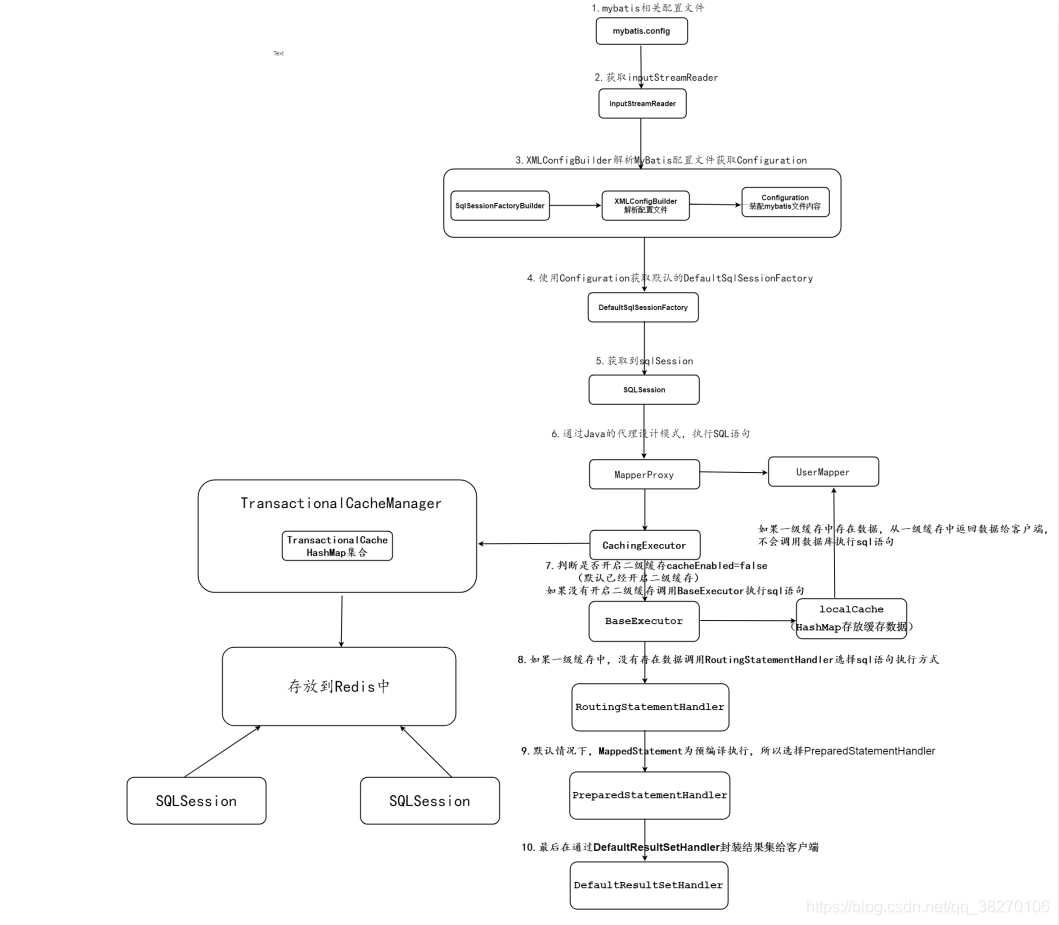
三、 注解的CRUD
1. 在MybatisUtils中开启自动提交
1 2 3
| public static SqlSession getSqlSession(){ return sqlSessionFactory.openSession(true); }
|
2. 编写接口文件
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public interface UserMapper { @Select("select * from user") List<User> getUser();
@Select("select * from user where id = #{id}") User getUserByID(@Param("id") int id);
@Insert("insert into user(id,name,pwd) values(#{id},#{name},#{password})") int insertUser(User user);
@Delete("delete from user where id = #{id}") int delUserByID(@Param("id") int id);
@Update("update user set name=#{name},pwd=#{password} where id = #{id}") int updateUser(User user); }
|
3. 编写测试类
1 2 3 4 5 6 7 8 9 10
| public static void main(String[] args) { SqlSession sqlSession = MybatisUtils.getSqlSession(); UserMapper mapper = sqlSession.getMapper(UserMapper.class); User user = mapper.getUserByID(2); System.out.println(user); mapper.insertUser(new User(6,"hello","1231234")); mapper.delUserByID(6); mapper.updateUser(new User(10,"hello","22223")); sqlSession.close(); }
|
关于@Param()
- 基于类型的参数或者String类型,需要加上。
- 引用类型不需要加。
- 如果只有一个基本类型的话,可以忽略,但是建议加上。
- 在SQL中引用的就是@Param()中设置的属性。
#{} 和 ${}区别
#{} 是预编译处理,像传进来的数据会加个" "(#将传入的数据都当成一个字符串,会对自动传入的数据加一个双引号)
${} 就是字符串替换。直接替换掉占位符。$方式一般用于传入数据库对象,例如传入表名.
使用 ${} 的话会导致 sql 注入。